Introduction to Php
In this article, we will talk about the PHP programming language. We will cover the basic syntax, variables, constants, data types, and more.
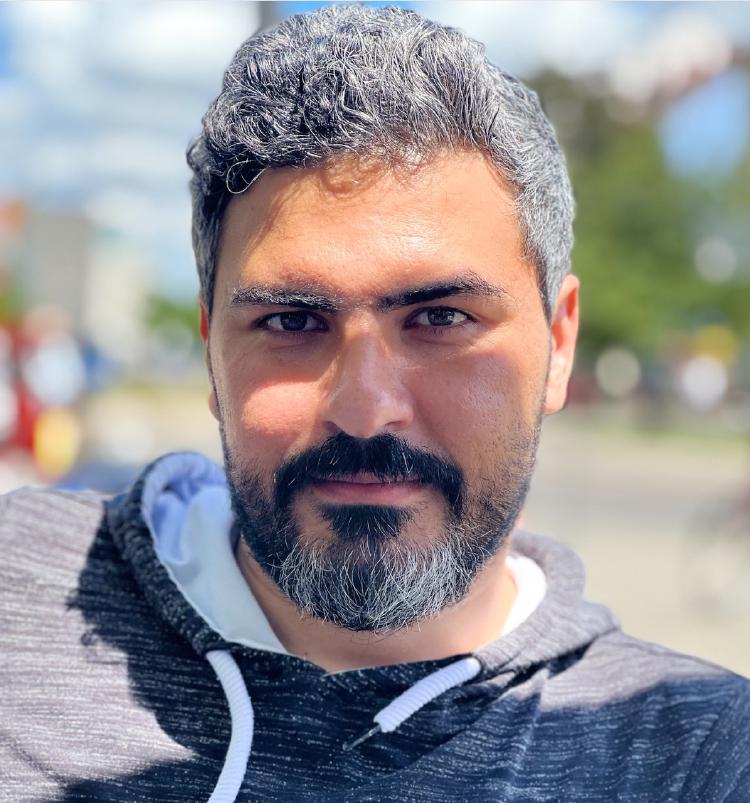
- Hassan Salem
- 16 min read
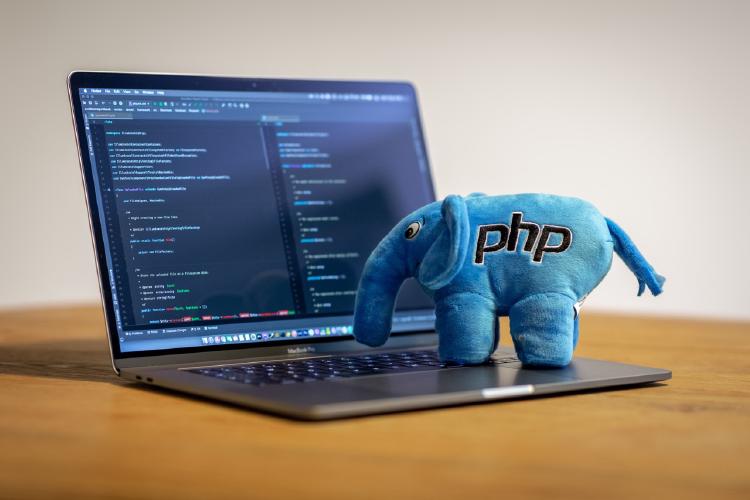
Basic PHP syntax
PHP file should start with the opening tag <?php
, and you can end it with the PHP closing tag ?>
. It is not recommended to add a closing tag if the file only contains PHP.
Every instruction in PHP should be terminated with a semicolon ;
<?php
echo 'Hello world';
Comments
Comments are lines in the code that will not be executed as a part of your application. These lines are only for other developers or code readers to understand the code.
There are many ways to write PHP comments, and PHP supports C
, C++
, and Unix style
comments.
Example:
// This is a single-line comment
# this is also a single-line comment
/**
This is
a multiline comment
*/
echo /** inline comment */ "hi";
// The above line will print "hi" and will ignore the comment
usually, your code should be expressive and should not need comments. So try to use the comments to describe why you wrote that code that way instead of what your code is doing! unless your code is a mess and you cannot fix it now, it is better to add comments describing what is happening.
echo
Echo is one of the PHP language construct, and its purpose is to output one or more strings that it takes as varargs. And it does not have any return type, so it cannot be used in an expression.
example of echo
usage:
<?php
echo('string1', 'string2');
// or
echo 'string1', 'string2';
print
Print is another language construct, and it does the same job as echo
, but it can only print one string at a time, and it will always return a value of 1.
example of print
usage:
<?php
print 'String';
// or
print('String');
PHP Variables
A variable is a symbol that stands for a value that can be changed later. For example, in PHP, we can define variables by having the $
concatenated with some words. E.g.: $name = 'Oliver Queen';
in our example, $name
is the variable and Oliver Queen
is the value that the variable stands for.
Some rules for PHP variables
- The variable should start with the
$
sign. - PHP variables are case-sensitive. It means that $x is not the same as $X.
- A valid PHP variable must start with a letter or underscore, followed by any letter or number or underscore.
$this
variable is a special variable that cannot be assigned
PHP variables are by default assigned by value, which means that if you assign anything to a variable, the whole thing will be copied into that variable. But you can also assign a variable by reference, which means that the value will not be copied, but the new variable will act as an alias of the old one. And you can achieve this by using the &
before the variable.
For example passing by value:
<?php
$name = 'Oliver Queen';
$oliver = $name;
// $name and $oliver have the same value now, which is 'Oliver Queen' for both of the vars
// If I change the $name variable value to 'John Diggle'
// The $oliver variable should not be affected
$name = 'John Diggle';
echo $name, $oliver;
// The result will be: Oliver Queen John Diggle
For example passing by reference:
<?php
$name = 'Oliver Queen';
$oliver = &$name;
// $name and $oliver are both variables, but $oliver is an alias for $name
// So if I change any of them, the other will be changed as well
$name = 'John Diggle';
// If I print both of them:
echo $name, $oliver;
// The result will be: John Diggle John Diggle
PHP Constant
Like the variable, a constant is a symbol or name that stands for a value, but the difference is that the content of this constant cannot be changed after it got assigned. So we use constants wherever there is data that should not be changed.
All variable rules also apply for the constant, but it doesn’t have to have the $
sign at its beginning.
Example:
<?php
const NAME = 'Oliver queen';
// NAME constant cannot be changed at execution time, and trying to change it will
// lead to an exception
Another way to define a constant is by using the define
function. But define
function cannot be used in a class definition. It should always be outside any class definition.
const
constant definition can be used inside and outside a class definition
define example:
<?php
// First argument is the name of the constant, and the second one is the value.
define ('A_NAME', 'Oliver Queen');
// And you can check if a const is already defined, we can use the `defined` function
// That will return true if the const is already defined and false if not
print defined('A_NAME'); // Will print 1
You can define a constant with all lower case letters, and that is acceptable, but the convention is to use all upper cases when defining constants in PHP.
Note that before PHP-7.3, you can define a case insensitive constant using
define
, which is not recommended and removed in PHP-8.
Const vs Define!
Both define
and const
can be used to define a constant, but there are some differences between them. So we, Will, start from the most to the least important.
const
defines the constants at compile time whiledefine
defines the constants at runtimedefine
cannot be used in a class definition while const can ** ```PHPdefine
can be used in a control structure while const cannot
PHP data types
We talked about variables and that they hold some data. In PHP, every piece of data has a type like 'Oliver Queen'
is a string, and 1
is an integer. The type of variables in PHP is usually decided at runtime by PHP, depending on the stored data.
Types
Php supports ten primitive types categorized as followed:
Scalar types
bool
A bool is true
or false
<?php
$isRunning = true;
$isRunning = false;
Control structures like conditionals and loops use boolean data types. In PHP, types can be evaluated to boolean either manually or automatically by the control structures.
<?php
$x = (bool)0; // evaluates to false
$x = (bool)-0; // evaluates to false
$x = (bool)0.0; // evaluates to false
$x = (bool)-0.0; // evaluates to false
$x = (bool)''; // evaluates to false
$x = (bool)null; // evaluates to false
$x = (bool)[]; // evaluates to false
$y = (bool)1; // evaluates to true
$y = (bool)1.1; // evaluates to true
$y = (bool)'Any non empty string'; // Evaluates to true
$y = (bool)'false' // evaluates to true because it is non empty string
$y = (bool)[1, 2, 3] // evaluates to true because it is non empty array
int
Int can be any whole numbers like: 1
, 2
, -3
and so on. You can find the minimum and maximum integer numbers by using the predefined constants PHP_INT_MIN
and PHP_INT_MAX
In PHP, you can define integers in multiple ways lie:
<?php
$age = 33; // decimal 33
$age = 0x21; // Is 33 in hex, when prefixed with `0x`
$age = 041; // Is 33 in octal, when prefixed with `0`
$age = 0b100001; // Is 33 in binary, when prefixed with `0b`
And for readability, you can use _
to separate the integer with underscores, so if you have 1000000000 you can define it like: $price = 1_000_000_000;
and PHP will evaluate it as 1000000000
float
Float can be any real number like: 1.0
, 2.2
, -2.88
and so on. As for the int, you can get the min and max values of float numbers by using the constants PHP_FLOAT_MIN
and PHP_FLOAT_MAX
Example:
<?php
$isGood = true; // Will be of type boolean
$name = 'Oliver Queen'; // Will be of type string
$age = 33; // Will be of type int
$price = 99.99; // Will be of type float
string
The string can be any text value like 'Any text'
. In PHP, a string should be enclosed by either single quote or double quotes like: $name = 'Oliver';
or $name = "Oliver";
. The difference between single and double quotes is that with double quotes, you can use available variables example:
<?php
$name = 'Oliver';
$fullName = "{$name} Queen";
echo $fullName; // Will print Oliver Queen
$fullName = '{$name} Queen';
echo $fullName; // Will print {$name} Queen
Heredock & Nowdoc
Another way to represent a string is using Heredoc and Nowdoc, and usually, these are used when you have a multiline string. This can be achieved by a special syntax, which is <<<
plus an identifier that could be anything, for example, if you are writing an SQL query, then you can use SQL
so your string will start with: <<<SQL
and end with SQL;
and if I’m printing HTML I would have something like <<<HTML
and ends with HTML;
Heredoc
<?php
$isBestFriend
// can use variables inside like the double quote strings
$query = <<<SQL
Select * from friends where is_best = $isBestFriend;
SQL;
Nowdoc: we just inclose the identifier with single quotes
<?php
// We cannot use variables inside Nowdoc
$query = <<<'SQL'
select * from friends where is_best = true;
SQL;
String concatination
You can concatenate two strings using the .
notation.
Example
<?php
$firstName = 'Oliver';
$lastName = 'Queen';
$fullName = $firstName . ' ' . $lastName;
echo $fullName; // Will print: Oliver Queen
echo "{$firstName} {$lastName}"; // Will print: Oliver Queen
Compound types
array
It can be a list of any value like a list of numbers, int, null, resource, or any type.
Array simple example:
$names = array('Oliver', 'John', 'Thea');
The shorthand of the
array()
method is the usage of the square brackets[]
. That means thatarray(1, 2, 3)
is identical to[1, 2, 3]
. For the rest of this book, we will only use square brackets.
In PHP, there are two kinds of arrays, associative and indexed arrays.
Indexed arrays: Are zero-based index array
Associative arrays: Are a key-based index
Example:
$indexedArray = [
'A', 'B', 'C', 'D'
];
$associativeArray = [
'color' => 'Red',
'price' => 2000
];
You can treat PHP arrays as a list, map, collection, queue, stack, and more.
Array access
Indexed array access:
To access an element in an indexed array, you have to use the index of that element. So, for example, if we have $array = ['A', 'B', 'C'];
and you want to access the B
you have to use the index of B
, which is 1
in our case because first element index starts with zero. And to get the element at index 1 of our array, we have to use the array access syntax, which looks like: $array[1]
the value of $array[1]
will be equal to B
.
$array = ['A', 'B', 'C'];
$array[0]; // Will be equals to A
$array[1]; // Will be equals to B
$array[2]; // Will be equals to C
Associative array access
Same as an indexed array, to access an associative array, you need to know the key of the element that you want to access in that array. But in associative arrays, the key is a string value instead of numeric. $map = ['color' => 'Red', 'price' => 2000]
. To access the Red
value, you have to know its key, color
in our case. So $map['color']
will have the value of Red
.
$map = ['color' => 'Red', 'price' => 2000];
$map['color']; // Will be equal to Red
$map['price']; // Will be equal to 2000
Array mutation
To mutate an array means to add, change, or delete some of its elements. In PHP, you can use the same square bracket syntax []
to modify array elements in both associative and indexed kinds of arrays.
$array = ['A', 'B', 'C'];
// You can replace the first element to be small letter a
$array[0] = 'a'; // The array will now be equal to ['a', 'B', 'C']
$map = ['color' => 'Red', 'price' => 2000];
// You can change the color value to 'Green'
$map['color] = 'Green'; // The array will now be equal to ['color' => 'Green', 'price' => 2000]
Adding an element to an indexed array
$array = ['A', 'B', 'C'];
// To add D to the array
$array[] = 'D'; // The array will be equal to ['A', 'B', 'C', 'D']
Adding an element to an associative array
For an associative array, you have to know which key you want to add to that array. So if we have this array $map = ['color' => 'Red']
and you want to add a price
, then you have to decide on the key, in our case, it is price
so to add an element you should use $map['price'] = 2000
.
Delete array element
To delete an element in both kinds of arrays, you can use the PHP function unset
. This function will destroy the variable you pass to it. In arrays, it will remove the element you give.
Example
$array = ['A', 'B', 'C'];
// To delete B
unset($array[1]); // the array will equal to: ['A', 'C']
object
As the array, it can hold more than one variable with different data types
- It can be an instance of either a built-in or user-defined class
- `subclass is a generic empty class that can be used to create generic objects
Basic implementation of PHP class would be:
class MyClass {
}
Then you can create objects of your class by using the new
keyword
$object1 = new MyClass();
callable
- By its name, callable is something that we can call like a function or an invokable class
iterable
** Iterable is a pseudo-type, and it means that the variable can be accessed as an array
Example:
<?php
$hobies = ['Reading', 'Video games']; // Will be array of strings
$things = ['A', 1, true, false, ['anything']]; // Will be an array of mixed types
$something = new stdClass(); // Will be of type object
$callable = function () { echo "HI :)"; }; // Will be of type callable
Special types
resource
- It can be any external resource like a file, database, or a stream
NULL
- This type is simple a variable with no value
Example:
<?php
$nothing = NULL; // Will be of type NULL
$resource = fopen('afile.txt'); // Will be of type resource
We can get the type of any variable by a special function
getType
so if we have a variable$x = 1
and we usegetType($x)
it will returninteger
. for more info you can visit getType doc
Type casting
In PHP, we can force a variable to be of any type. And casting can be achieved by adding the type name between parentheses in front of the variable you want to cast like: (type)$var
Example
<?php
$age = '22'; // This '22' is of type string
echo getType($age); // Will print string
$age = (int)'22';
echo getType($age); // Now it will print integer
Control structures
Are set of features that allows us to control the code execution. Or in other words, it allows your code to behave according to the input you provide.
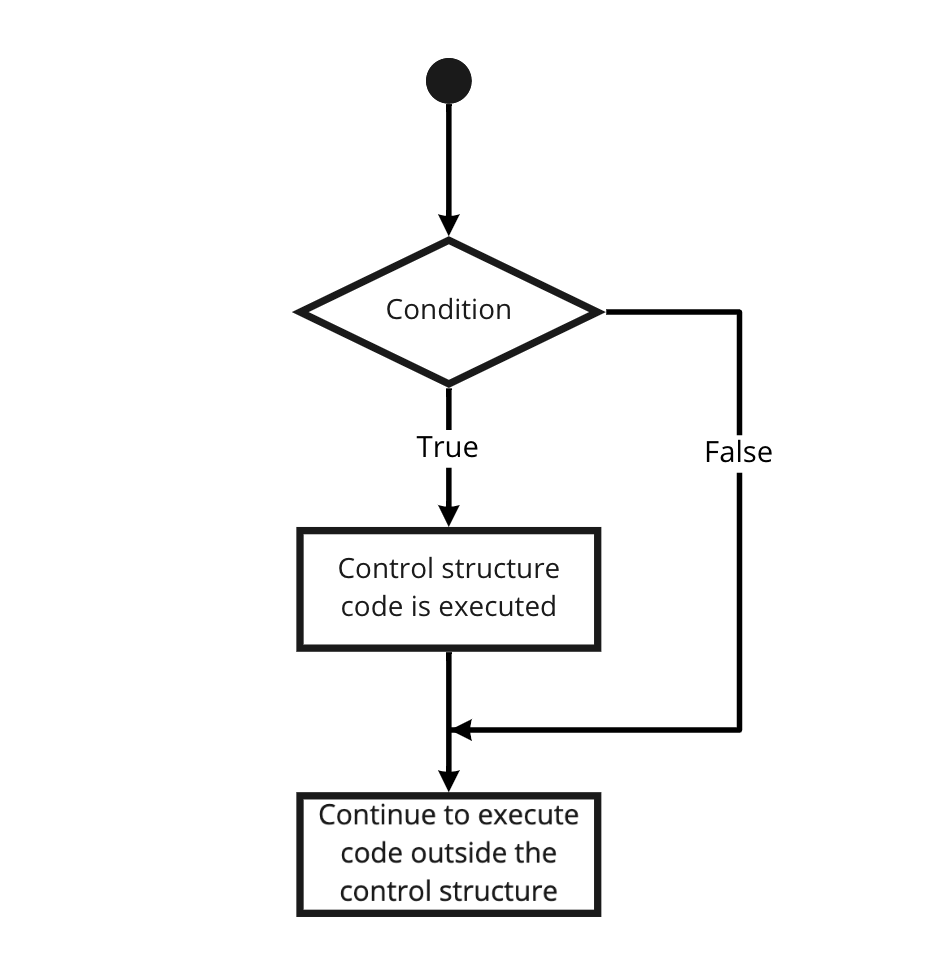
Loops
Loops are used to repeat a specific statement or code block, like you can print some string 100 times.
PHP supports four kinds of loops which are while
, do while
, for
, and foreach
. Every one of the loops has some specific usage and criteria.
while
While is very simple and it accepts one argument of type boolean we call it the loop expression. As its name hints: while the expression evaluates to true, it will keep executing the code inside its body
Example of php while loop
<?php
while (true) {
echo "I love PHP";
}
The above example is a very simple infinite loop using while
. The code will keep printing I love PHP
until we terminate it manually because the loop condition is always true.
Animation that illustrates how the while loop works
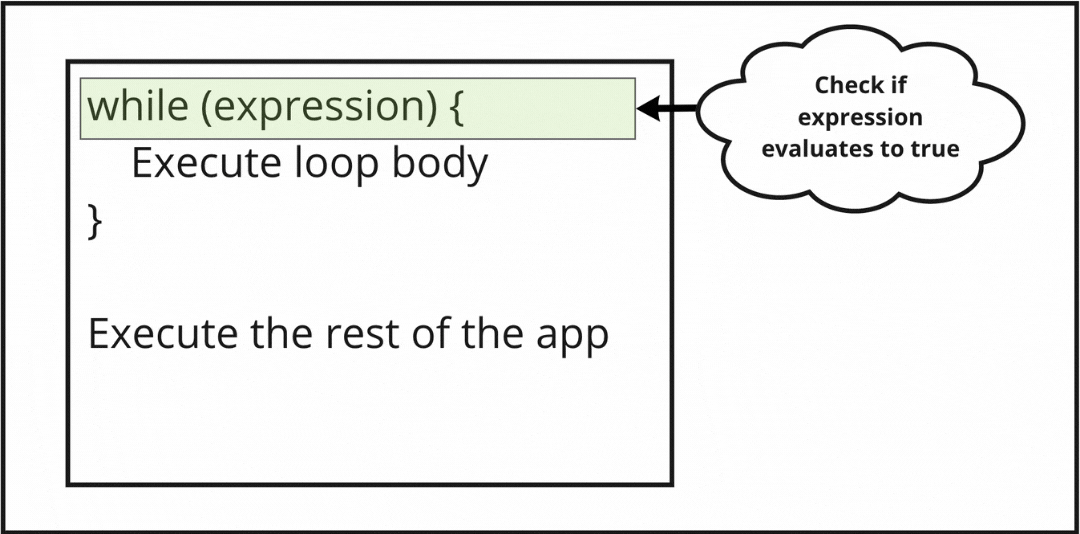
do-while
Do while works exactly as the while
loop but with one difference that it checks the expression if it is true or false at the end of the iteration instead of the begining of it.
So even if the condition expression is false, the loop will execute the body at least once.
Example
<?php
do {
echo "I love PHP";
} while ($someCondition);
for
For loop is not as simple as the while
and its syntax differs as well. The for loop accepts 3 expressions: The initial expression which executes only once at the beginning of the loop. The second one is the condition expression that executes before every iteration of the loop. The third one is the update expression which executes at the end of every iteration.
Animation that illustrates how the for loop works
A simple for loop example:
<?php
for($counter = 0 ; $counter < 100 ; $counter += 1) {
echo $counter;
}
- First step it will initialise
$counter = 0
- Then it will check if the condition expression evaluates to true. In our case
$counter
is less than 100, because it is0
for now. - then it will execute the body which will print
0
- Then it will execute the update expression
$counter ++
<- this will increment the$counter
variable by1
and now it is equal to1
. - Then it will check the condition and still
1
is less than100
- If condition less than
100
go to step3
, else exit the loop
foreach
Foreach is a special for loop that is mainly used to iterate over an array and objects. And it provides access to the current.
Thre is no condition expression with foreach, it will keep iterating over the array or object until the last element reached.
Basic foreach
example:
<?php
$array = ['A', 'B', 'C'];
foreach($array as $arrayElement) {
echo $arrayElement;
}
Another example with element key usage
<?php
$car = [
'color' => 'Red',
'made_in' => 'Germany',
'brand' => 'Mercedes Benz',
];
foreach($car as $key => $value) {
echo "$key is $value\n"; // \n is just for printing a new line
}
// The result will be:
/**
color is Red
made_in is Germany
brand is Mercedes Benz
*/
foreach
can also be used to iterate over an object attributes values.
A very important note that the variable that you introduce by the foreach loop stays thrughout the application execution. In our last example the $value
variable will stay and it will hold the latest value it iterated over. And worse if before the loop you had that variable, the foreach will change it to the latest value it iterated over.
This behaviour can lead to many unexpected behavior and will end up with some bugs.
Example:
<?php
$value = 'ABC';
$array = [1, 2, 3];
foreach($array as $value) {
echo $value;
}
echo $value;
// This will not print 'ABC'. It will print 3
// Because 3 is the latest value the foreach iterated over.
it is recommended to unset the reference of the variable you created for the foreach.
<?php
foreach($array as $value) {
echo $value;
}
unset($value);
goto
🤦
The goto
operator also considered as a control structure and it can jump between the PHP script sections. And you can use it to easily create a complicated spaghetti code :). That is because goto
is a very easy to abuse statement.
Example:
<?php
echo "1";
goto last;
echo "2";
echo "3";
last:
echo "4";
The above code will print: 14
goto
considered bad practice and it should be avoided, some developers suggest that it can be used to break a deep nested loops but in my opinion if we allow it once then we will lose control later and we will find it in many places in our code.
Lets forget about it :D
Conditionals
This group of statements used to conditionally execute a code fragment. There are multiple kinds of conditional statements like if
, else
, elseif
, switch
, and match
.
if
if
is one of the most important features in programming and it can be used to allow the software to make some decision based on some condition.
<?php
if($someExpression) {
echo "I love PHP";
}
The above code will print I love PHP
only if the $someExpression
evaluates to true.
Animation of a basic if statement: