You Think You Know Php
PHP is a widely-used open source general-purpose scripting language that is especially suited for web development and can be embedded into HTML.
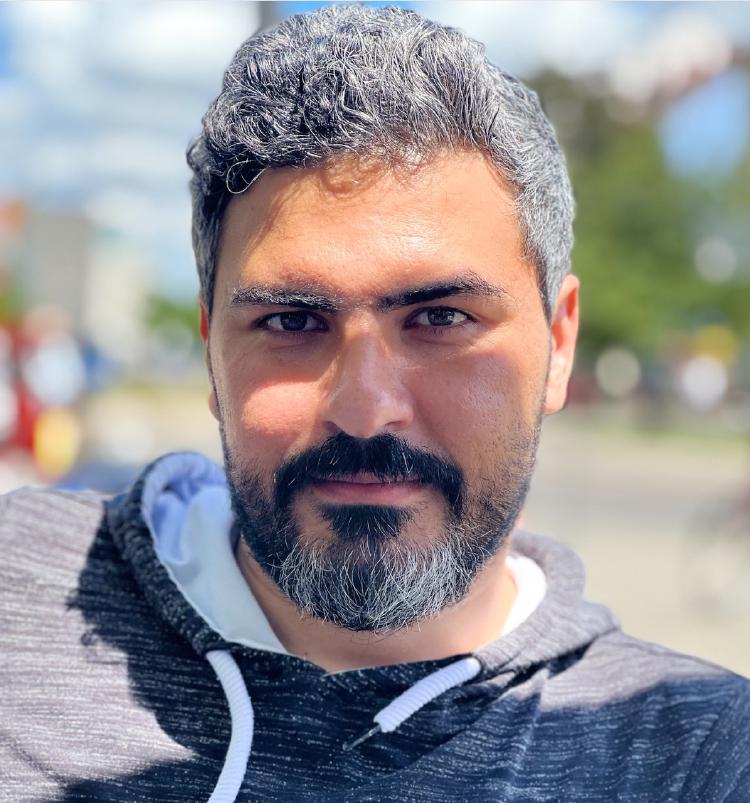
- Hassan Salem
- 5 min read
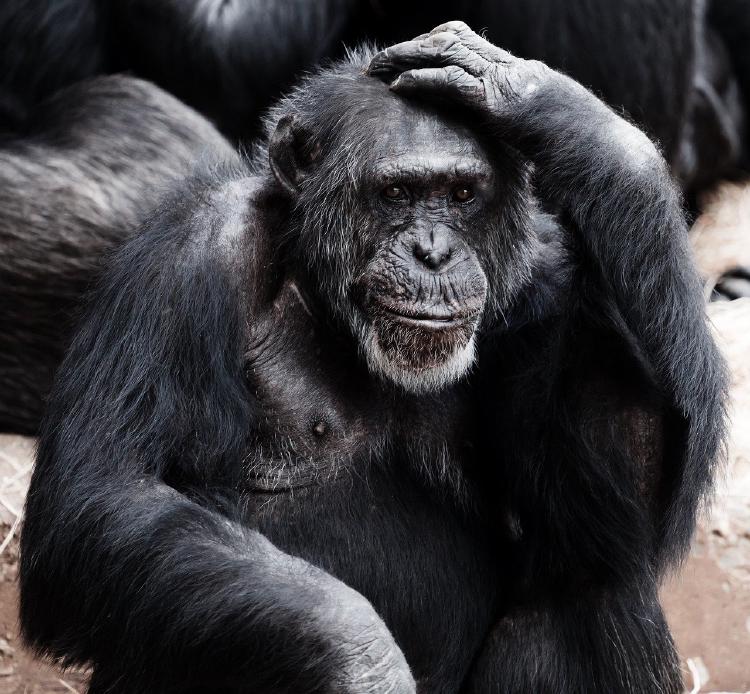
PHP (recursive acronym for PHP: Hypertext Preprocessor) is a widely-used open source general-purpose scripting language that is especially suited for web development and can be embedded into HTML.
Many developers around the globe have started their careers with PHP, and a lot of them still do. PHP powers 79% of websites on the internet. The popularity of this language is because you don’t need a lot of experience to write a well-working software with it and because many famous platforms are built on top of PHP like WordPress, Joomla, and Drupal.
If you are already working with PHP, you know what I’m talking about. And I think you think you know PHP. While I’m sure you know many things about PHP and are a good developer, some topics are less known than others.
Increment a string
Almost every developer increment a number at least once in their career, like having a variable with a numeric value, and you increment/decrement it. example:
<?php
$counter = 0; // Variable initialization
$counter++; // Post-increment
$counter--; // Post-decrement
++$counter; // Pre-increment
--$counter; // Post-decrement
All the above examples will add 1 in case of increment and subtract 1 in case of decrement.
But what if that value has a string like “iPhone 5”? What would the result be?
<?php
$modelName = 'iPhone 1';
$modelName++;
echo $modelName; // Will print: "iPhone 2"
Just note that “decrement” does not have any effect on strings. It only works on numbers. And “increment” does not affect spaces in a string.
Then what will be the result of incrementing many times? Let’s say ten times would it keep counting up forever?
No, in our example, the result will go from “iPhone 1” to “iPhone 9” and loop back to “iPhone 0” and do the same over and over. And that is because there is a space before the number. But if we want it to go up to 99, we need to have the value in this format: iPhone 01
, which will loop numbers from 0 to 99. But what if we remove the space? like “iPhone1”? This will increment everything starting from right to left when it reaches 9. Then it will increment the “e” which will give us “f” and will see “iPhonf0”
<?php
$modelName = "iPhone9";
echo ++$modelName; // Will print: "iPhonf0"
echo ++$modelName; // Will print: "iPhonf1"
For more information about PHP increment, please visit the docs
in_array
is not what you think
In_array indicates that it checks if an item is in an array, which is true, but you should be careful how to use this function. There are two ways you can check if an item exists in an array with this function, the first one is strict
check, and the second one is nonstrict
check, but that is not so clear from the function name, so many people will use it without the strict flag which might lead to unexpected results.
Example:
$array = ['first element', 'second element'];
var_dump(in_array(0, $array));
the previous code will print true
Another example:
$array = ['1 apple', '2 oranges'];
var_dump(in_array(1, $array));
var_dump(in_array(2, $array));
Guess what?! both will print true
The last example:
$array = ['any thing', '0 oranges'];
var_dump(in_array(true, $array));
var_dump(in_array(false, $array));
And yes, you are correct. It will print true! But why? PHP has two types of comparison. One is strict that uses three equals ===
, the second is not strict which uses double equals operators ==
. There are many differences between both, but the most important one is that strict PHP will first compare the types of both operands, and their types should be the same to consider operands equal. The non-strict comparison will cast the string to a numeric type if the comparison is with a number. So that is why you should always use the strict mode with in_array
. More information about in_array
please take a look at PHP in_array docs
ArrayAccess interface will allow your object to behave as an array but won’t work with array functions!
I think this is more of a weird implementation. If you have an object that implements the ArrayAccess
interface, you will be able to access its values as if it is an array, but you will not be able to use array functions like in_array
on it. Instead, you will get an exception that the function expects an array, but object given.
Can’t throw exceptions from __toString() functions
Before PHP 7.4, it was impossible to throw exceptions from the __toString
function, and you will get a fatal error if you do so.
Objects are passed by reference. No
I hear that a lot, that objects are passed by reference. Maybe because if you passed an object to a function that changes it, then the original one will be changed as well. Although the result is the same, PHP objects aren’t passed by reference.
A PHP reference is an alias, allowing two different variables to write to the same value.
And in PHP, if you have a variable that contains an object, that variable does not contain the object itself. Instead, it contains an identifier for that object. The object accessor will use the identifier to find the actual object. So when we use the object as an argument in function or assign it to another variable, we will be copying the identifier that points to the object itself.
An elementary example would be:
class Type {}
$x = new Type();
$y = $x;
$y = "New value";
var_dump($x); // Will print the object.
var_dump($y); // Will print the "New value"
$z = &$x; // $z is a reference of $x
$z = "New value";
var_dump($x); // Will print "New value"
var_dump($z); // Will print "New value"
Final words
PHP is a very old language, and it got evolved a lot over time. As a result, it has many features, some of them are popular, and some are not. If you know another unpopular feature/idea about PHP, please let us know in the comments or contact me directly @Salem_hsn